Usage examples for Python
Get and show an image
Following python script is the most simple usage example of
the API to get and show an image. Each method’s default value
is used if user don’t input as argument. If you got ssl error,
please set ssl_verify to False
.
# Load module
from jaxa.earth import je
# Get an image
data = je.ImageCollection(ssl_verify=True)\
.filter_date()\
.filter_resolution()\
.filter_bounds()\
.select()\
.get_images()
# Process and show an image
img = je.ImageProcess(data)\
.show_images()
A Following image will be shown.
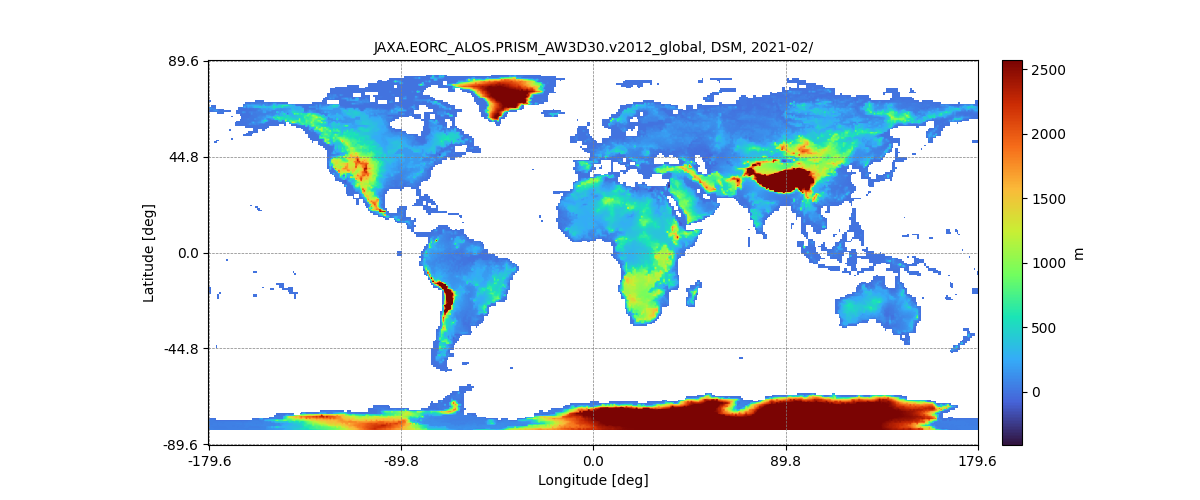
If you would like to get raw numpy array and show it, please execute following script.
import matplotlib.pyplot as plt
plt.imshow(img.raster.img[0])
plt.show()
A Following image will be shown.
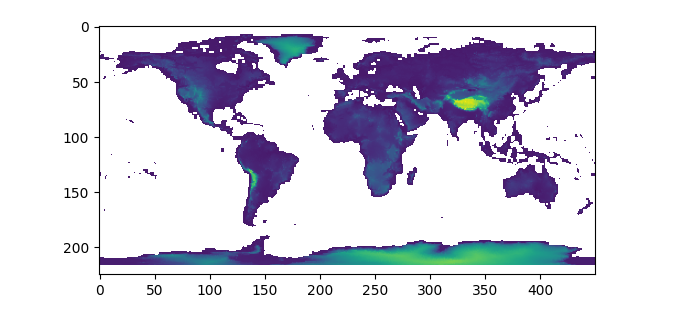
Search collection’s ID and Band
If you would like to know all collection’s ID and Band, please check the page.
https://data.earth.jaxa.jp/en/datasets/
By putting a list of keywords into a method filter_name
of the class ImageCollectionList
,
you can easily search and identify the formal ID and band of the collection.
Get and show an image by region of interest
Next, set each parameters such as kw (keyword to detect collection from collection’s id), dlim (date limit), ppu (pixels per unit(one degree)).
Set region of interest by bbox
If you set bbox (bounding box), requested area will be shown.
# Load module
from jaxa.earth import je
# Set query parameters
kw = ["Aqua","LST","half-monthly"]
dlim = ["2021-01-01T00:00:00","2021-01-01T00:00:00"]
ppu = 5
bbox = [110, 20, 160, 50]
# Get information of collections,bands
collections,bands = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw)
# Get an image
data = je.ImageCollection(collection=collections[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(bbox=bbox)\
.select(band=bands[0][0])\
.get_images()
# Process and show an image
img = je.ImageProcess(data)\
.show_images()
A Following image will be shown.
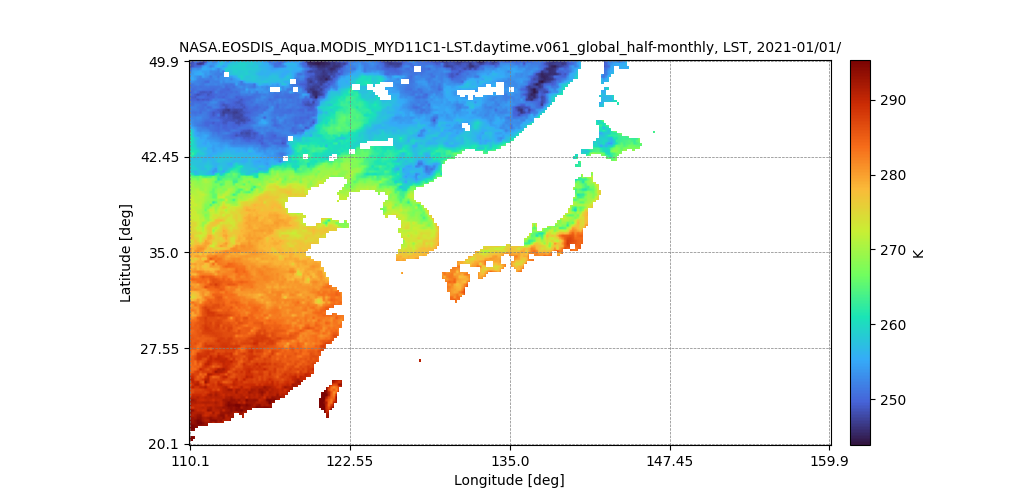
Set region of interest by geojson
If you read and set geojson data from your computer, requested area will be shown. User can create geojson data in web service (geojson.io) or convert from other vector format such as shape file in QGIS.
# Load module
from jaxa.earth import je
# Set query parameters
kw = ["Aqua","LST","half-monthly"]
dlim = ["2021-01-01T00:00:00","2021-01-01T00:00:00"]
ppu = 20
# Get information of collections,bands
collections,bands = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw)
# Get feature collection data
geoj_path = "test.geojson"
geoj = je.FeatureCollection().read(geoj_path).select()
# Get an image
data = je.ImageCollection(collection=collections[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(geoj=geoj[0])\
.select(band=bands[0][0])\
.get_images()
# Process and show an image
img = je.ImageProcess(data)\
.show_images()
A Following image will be shown.
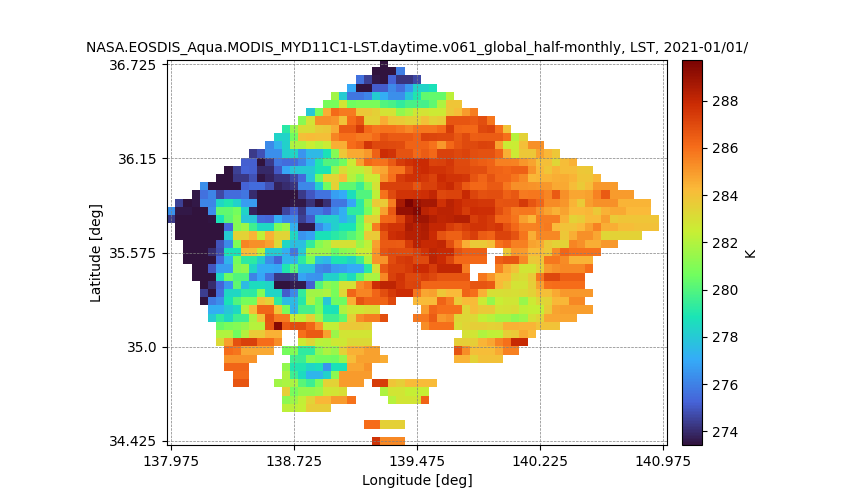
Get and show a masked image
Next, let’s use ImageProcess
class object’s method mask_images
to
mask data. Two kind of data (mask and data to be masked) is need to be aquired.
Extract range data pixels
In this example, GSMaP (Monthly Rain rate) is used as data, AW3D DSM (Elevation) is used as masking. GSMaP data is extracted by AW3D’s range from 0.01 to 1000.
# Load module
from jaxa.earth import je
# Set query parameters
kw_d = ["GSMaP","monthly"]
kw_m = ["AW3D"]
dlim = ["2021-02-01T00:00:00","2021-02-01T00:00:00"]
ppu = 10
bbox = [110, 20, 160, 50]
mq = "range"
val = [0.1,1000]
# Get information of collections,bands for data
collections_d,bands_d = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw_d)
# Get an image for data
data_d = je.ImageCollection(collection=collections_d[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(bbox=bbox)\
.select(band=bands_d[0][0])\
.get_images()
# Get information of collections,bands for mask
collections_m,bands_m = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw_m)
# Get an image for mask
data_m = je.ImageCollection(collection=collections_m[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(bbox=bbox)\
.select(band=bands_m[0][0])\
.get_images()
# Process and show an image
img = je.ImageProcess(data_d)\
.mask_images(data_m,method_query=mq, values=val)\
.show_images()
A Following image will be shown.
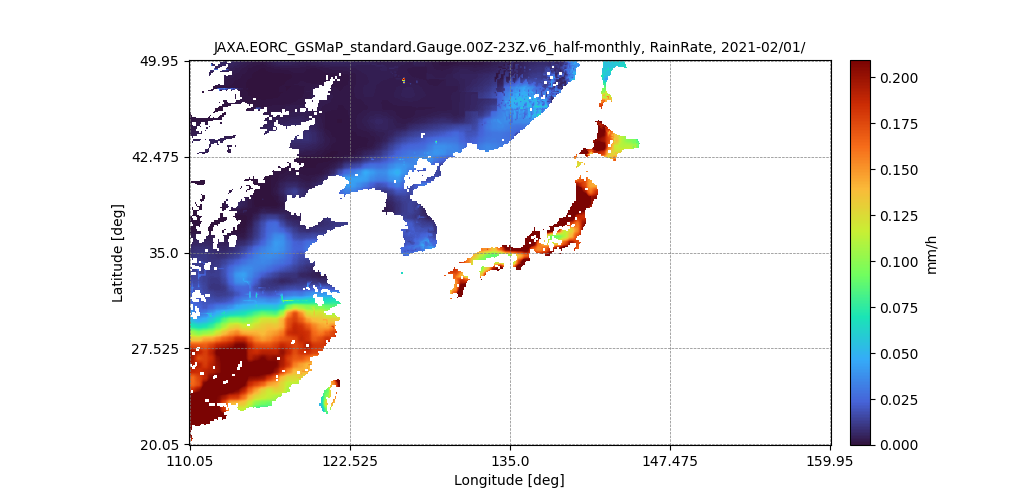
Extract values equal data pixels
In this example, AW3D DSM (Elevation) is used as data, LCCS (Land cover) is used as masking. AW3D data is extracted by LCCS values of 190 (Urban areas).
# Load module
from jaxa.earth import je
# Set query parameters
kw_d = ["AW3D"]
kw_m = ["LCCS"]
dlim = ["2019-01-01T00:00:00","2021-02-01T00:00:00"]
ppu = 360
bbox = [139.5, 35, 140.5, 36]
mq = "values_equal"
val = [190]
# Get information of collections,bands for data
collections_d,bands_d = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw_d)
# Get an image for data
data_d = je.ImageCollection(collection=collections_d[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(bbox=bbox)\
.select(band=bands_d[0][0])\
.get_images()
# Get information of collections,bands for mask
collections_m,bands_m = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw_m)
# Get an image for mask
data_m = je.ImageCollection(collection=collections_m[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(bbox=bbox)\
.select(band=bands_m[0][0])\
.get_images()
# Process and show an image
img = je.ImageProcess(data_d)\
.mask_images(data_m,method_query=mq, values=val)\
.show_images()
A Following image will be shown.
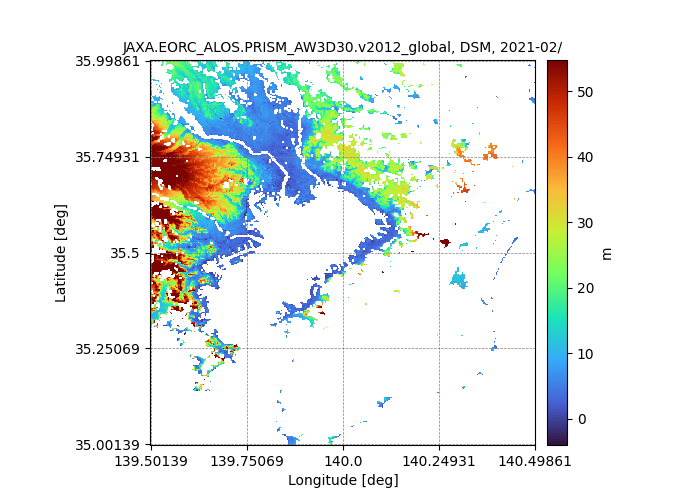
Extract bits equal data pixels
In this example, AW3D DSM (Elevation) and MSK(Mask) is used as data and mask. AW3D data band has two bands such as DSM(Elevation) and MSK (Mask). DSM is extracted by MSK bits of all zero (effective pixels).
# Load module
from jaxa.earth import je
# Set query parameters
kw = ["AW3D"]
dlim = ["2019-01-01T00:00:00","2021-02-01T00:00:00"]
ppu = 360
bbox = [139.5, 35, 140.5, 36]
mq = "bits_equal"
val = [0,0,0,0,0,0,0,0]
# Get information of collections,bands for data
collections,bands = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw)
# Get an image for data
data_d = je.ImageCollection(collection=collections[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(bbox=bbox)\
.select(band="DSM")\
.get_images()
# Get an image for mask
data_m = je.ImageCollection(collection=collections[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(bbox=bbox)\
.select(band="MSK")\
.get_images()
# Process and show an image
img = je.ImageProcess(data_d)\
.mask_images(data_m,method_query=mq, values=val)\
.show_images()
A Following image will be shown.
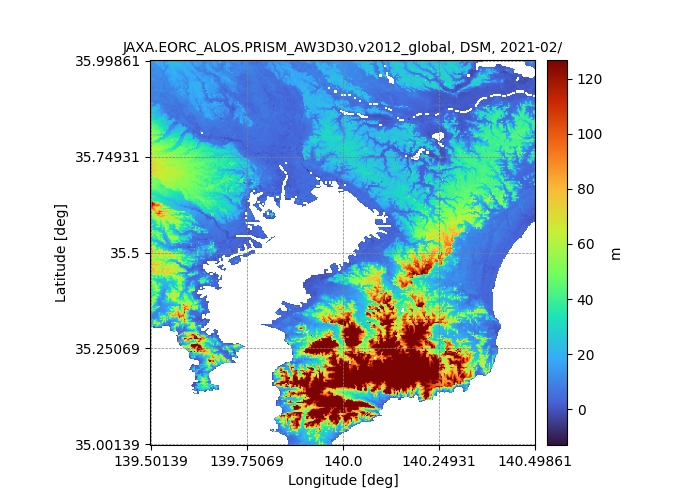
Get and show a differencial image
Next, let’s use ImageProcess
class object’s method diff_images
to
get differencial data. Two kind of data (data and reference) is need to be aquired.
In this example, NDVI (Aqua, monthly) is used as data, NDVI (Aqua, monthly-normal) is
used as reference.
# Load module
from jaxa.earth import je
# Set query parameters
kw_d = ["ndvi","_monthly"]
kw_r = ["ndvi","_monthly","normal"]
dlim = ["2021-08-01T00:00:00","2021-08-01T00:00:00"]
# Get information of collections,bands for data
collections_d,bands_d = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw_d)
# Get an image for data
data_d = je.ImageCollection(collection=collections_d[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution()\
.filter_bounds()\
.select(band=bands_d[0][0])\
.get_images()
# Get information of collections,bands for reference
collections_r,bands_r = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw_r)
# Get an image for reference
data_r = je.ImageCollection(collection=collections_r[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution()\
.filter_bounds()\
.select(band=bands_r[0][0])\
.get_images()
# Process and show an image
img = je.ImageProcess(data_d)\
.diff_images(data_r)\
.show_images()
A Following image will be shown.
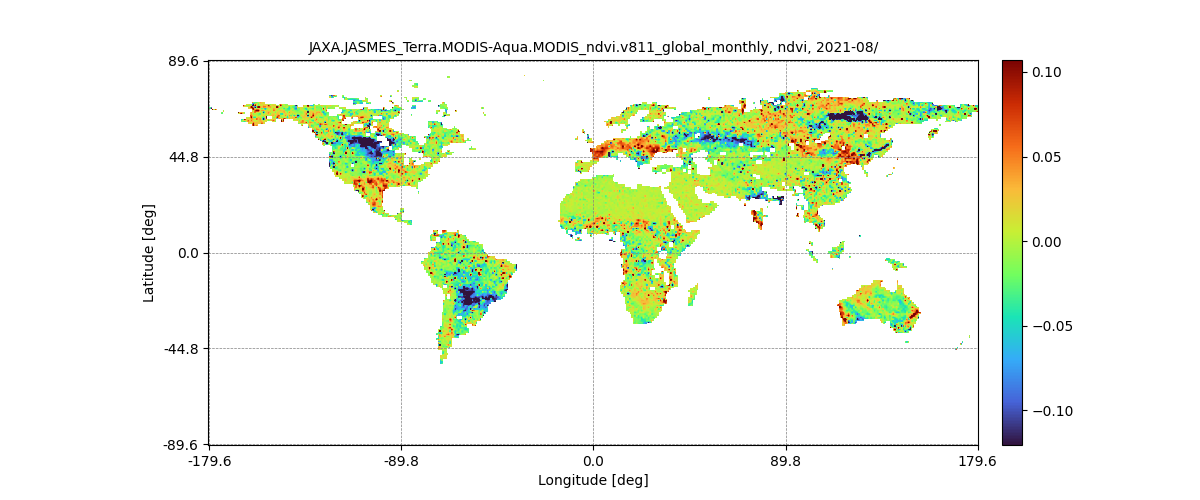
Get and show a composite image
Next, let’s use ImageProcess
class object’s method calc_temporal_stats
to
get a composite image. In this example, SMC (Soil Moisture Content, daily) is used
to be composite.
# Load module
from jaxa.earth import je
# Set query parameters
kw_d = ["SMC","_daily"]
dlim = ["2021-08-01T00:00:00","2021-08-10T00:00:00"]
# Get information of collections,bands for data
collections,bands = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw_d)
# Get an image for data
data = je.ImageCollection(collection=collections[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution()\
.filter_bounds()\
.select(band=bands[0][0])\
.get_images()
# Process and show an image
img = je.ImageProcess(data)\
.calc_temporal_stats("mean")\
.show_images()
A Following image will be shown.
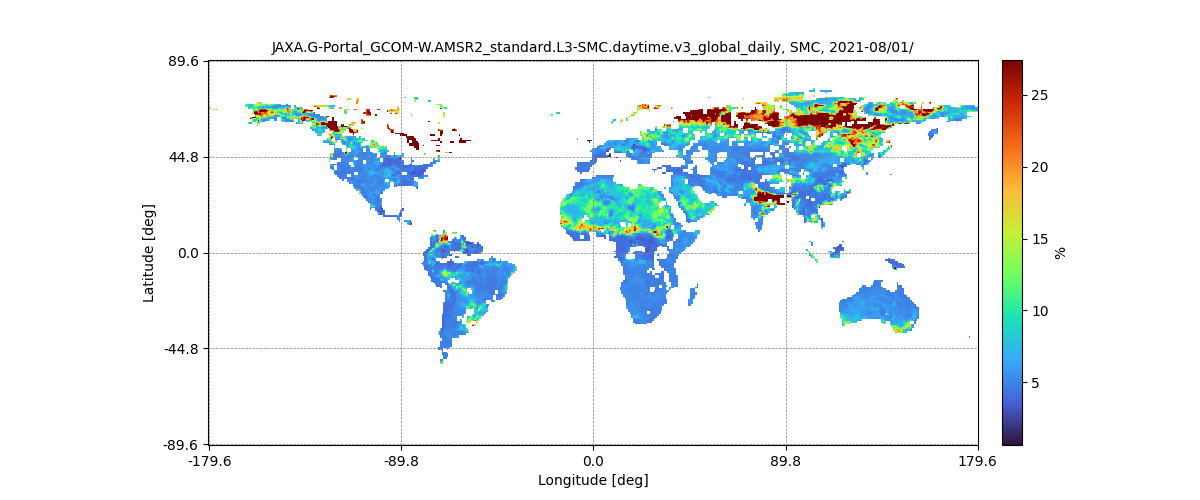
Caluculate and show timeseries data
Finally, let’s use ImageProcess
class object’s method calc_spatial_stats
and show_spatial_stats
to calclate and show spatial statistics.
In this example, swr (Short Wave Radiation, half-monthly-normal) is used.
# Load module
from jaxa.earth import je
# Set query parameters
kw_d = ["swr","half-monthly-normal"]
dlim = ["2021-01-01T00:00:00","2021-12-31T00:00:00"]
bbox = [120,20,150,50]
ppu = 10
# Get information of collections,bands for data
collections,bands = je.ImageCollectionList(ssl_verify=True)\
.filter_name(keywords=kw_d)
# Get an image for data
data = je.ImageCollection(collection=collections[0],ssl_verify=True)\
.filter_date(dlim=dlim)\
.filter_resolution(ppu=ppu)\
.filter_bounds(bbox=bbox)\
.select(band=bands[0][0])\
.get_images()
# Process and show an image
img = je.ImageProcess(data)\
.calc_spatial_stats()\
.show_spatial_stats()
A Following time series graph will be shown.
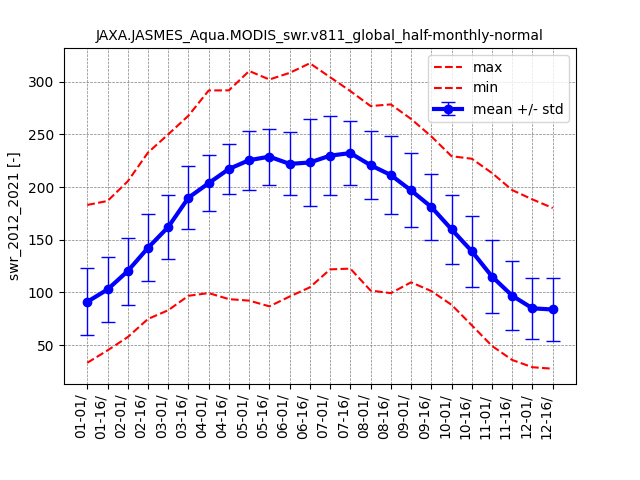